Implement 2D #31
Labels
No labels
Kind/Breaking
Kind/Bug
Kind/Documentation
Kind/Enhancement
Kind/Feature
Kind/Security
Kind/Testing
Priority
Critical
Priority
High
Priority
Low
Priority
Medium
Reviewed
Confirmed
Reviewed
Duplicate
Reviewed
Invalid
Reviewed
Won't Fix
Status
Abandoned
Status
Blocked
Status
Need More Info
No milestone
No project
No assignees
1 participant
Notifications
Total time spent: 8 hours
Due date
SeanOMik
8 hours
No due date set.
Dependencies
No dependencies set.
Reference: SeanOMik/lyra-engine#31
Loading…
Add table
Reference in a new issue
No description provided.
Delete branch "%!s()"
Deleting a branch is permanent. Although the deleted branch may continue to exist for a short time before it actually gets removed, it CANNOT be undone in most cases. Continue?
Currently, the engine is only 3D. I want to implement 2D since its easier to make art for, and I am no artist lmao. The renderer graph is hard coded by the
BasicRenderer::new()
function, I need to add a way to get the renderer and manually create the graph. This would make it more versatile and I could maybe create something likeRenderer2DPlugin
that constructs the render graph for 2D.I got a 2D sprite rendering!
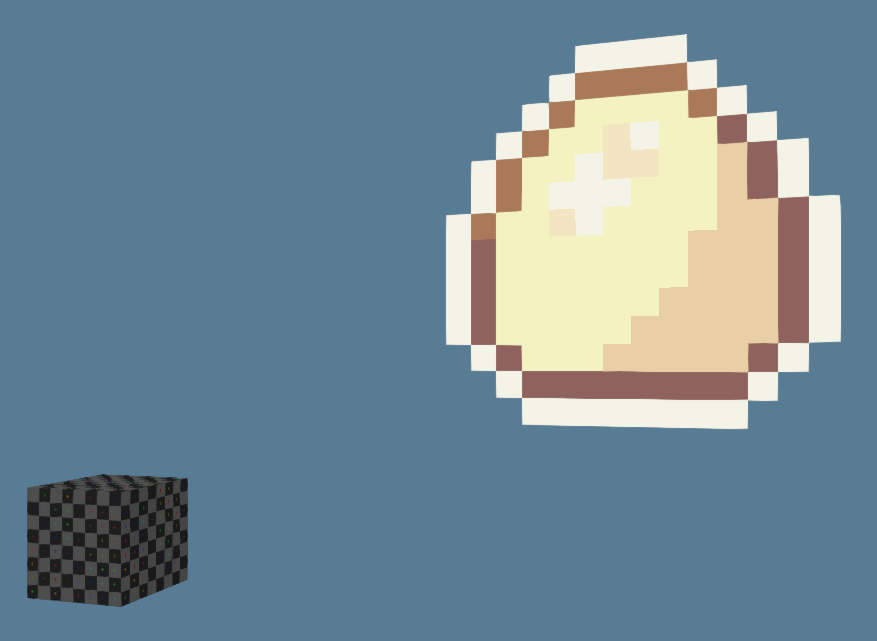
Its an egg from a free sprite pack I found on itch.io.
Since the Sprite rendering code is just a node in the render graph, I didn't have to do anything special to render 3d (the cube) at the same time.
Sprites are massive though since the dimensions of the images are used to create the quad, meaning that 1 pixel is the same as 1 unit. The cube in the above screenshot is a 1x1x1 cube, so each pixel in the egg texture is the size of the cube.
The camera movement when its projection is orthographic doesn't work very well at all. I need to take a look at that.
Not long after the last post, I implemented a working orthographic projection. The camera movement I talked about was actually issues with the projection (who would've expected lol).
I also implemented scaling modes for the projection, so you can set the size of the screen to fit a certain amount of world units. The above screenshot was taken with a fix height of 180 world units:
I still don't have a 2d camera controller implemented, still reusing the 3d one. It should be easy to write a top down controller, so I'll do that next.
The top down controller is complete! I also implemented zooming, but its kind of wonky when I have a fixed height scale mode on the projection since it doesn't zoom into the origin of the screen. I think its because the origin of the projection is not the origin of the screen. I'm not really sure how I would fix that yet...
The top down controller requires the system (
top_down_2d_camera_controller
) to be added to world, this can be done with theTopDown2dCameraPlugin
plugin, or you can manually add it. After its added, just add theTopDown2dCamera
component to the camera entity and configure its settings:The zoom can be disabled by setting the
zoom_speed
toNone
, then the max and min zoom can be whatever since they wont be used. Since the zoom is actually just modifying the scale parameter of the projection, the max is actually the minimum scale value. As the scale approaches zero, the camera appears to be more zoomed in.I implemented a
TextureAtlas
for using sprites from an atlas. I made a little demo that would create an entity with anAtlasSprite
(a sprite from an atlas) and animate it!This was done by a system that would manually move the "frame" of the sprite and wrapping it around when it needs to restart. Its hacky, I'll make something more general next.
Its pretty easy to load an atlas. This code example creates one using a loaded texture, and sets up the grid:
This isn't very useful for more unique atlases, so maybe I should make it just store
Vec<URect>
inside of it, then anew_grid
function that would automatically fill the vec with a grid of rects.Its now easier to animate sprites!
I created an
AtlasAnimations
component that stores multiple animations for a sprite in it. You can then useAtlasAnimations::get_active
to get anActiveAtlasAnimation
component that will define the current animation, and the position in it.Here's some code:
There's an ECS system that does the actual animation,
system_sprite_atlas_animation
.You can also insert a
ResHandle<AtlasAnimations>
on an entity to use as its animations. This lowers the amount of data being cloned and animations will likely be put on many entities. Updated code example:I've implemented a TileMap!
The tile textures in the map were randomly chosen using a weighted distribution. This is the code used to create the tile map:
Its pretty easy, even when using a weighted distribution. To make it easier to position things on the grid of the map, I created a
TileMapPos
component. You specify the entity that the tile map is spawned on, the x, y position on the tile map and the z-level. Here's some code that spawns eggs at random positions on the tilemap.There is a small issue where if the camera is moved, randomly lines between the tiles of the grid will appear. I think this is because of floating point precision, but I haven't looked into it yet. I think I would just remove a tiny amount of padding between the tile Transforms.
This was merged with #32